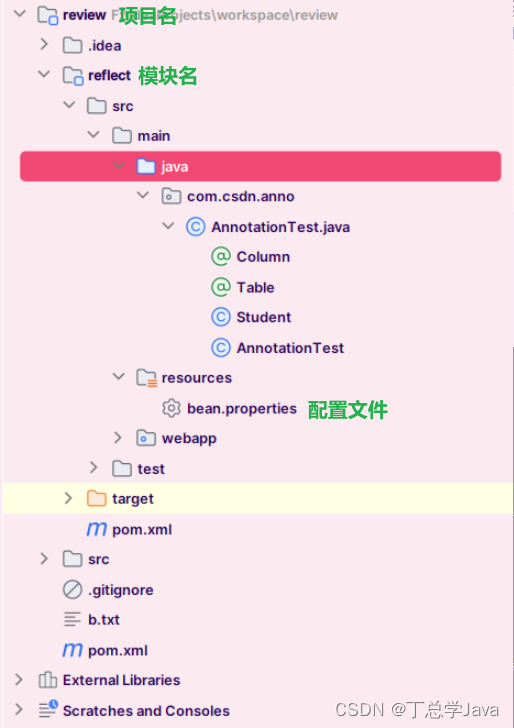
1、使用反射拼接SQL语句
package com.csdn.anno;
import java.io.IOException;
import java.io.InputStream;
import java.lang.reflect.Field;
import java.util.Properties;
public class AnnotationTest {
public static void main(String[] args) throws ClassNotFoundException, IOException {
// String sql = "select name,age,gender,city,score from student";
// StringBuilder sql = new StringBuilder("select XXX,XXX,XXX");
StringBuilder sql = new StringBuilder("select ");
//通过 类加载器 读取文件
InputStream inputStream = AnnotationTest.class.getClassLoader().getResourceAsStream("bean.properties");
System.out.println(inputStream);//java.io.BufferedInputStream@7cca494b
//读取properties配置文件,获取到配置文件里指定的类名
Properties prop = new Properties();
prop.load(AnnotationTest.class.getClassLoader().getResourceAsStream("bean.properties"));
String className = (String) prop.get("className");
//使用反射通过类名加载类
Class<?> clz = Class.forName(className);
String tableName = clz.getSimpleName().toLowerCase();
Field[] fields = clz.getDeclaredFields();
for (int i = 0; i < fields.length; i++) {
//遍历字段,获取字段名,添加到SQL语句
String name = fields[i].getName();
sql.append(name);
if (i!= fields.length-1) {
sql.append(",");
}
}
sql.append(" from ").append(tableName);
System.out.println(sql);//select name,age,gender,city,score from student
}
}
class Student {
private String name;
private int age;
private String gender;
private String city;
private String score;
}
className=com.csdn.anno.Student
2、使用 反射 + 注解 拼接SQL语句
package com.csdn.anno;
import java.io.IOException;
import java.io.InputStream;
import java.lang.annotation.Retention;
import java.lang.annotation.RetentionPolicy;
import java.lang.reflect.Field;
import java.util.Properties;
public class AnnotationTest {
public static void main(String[] args) throws ClassNotFoundException, IOException {
// String sql = "select name,age,gender,city,score from student";
// StringBuilder sql = new StringBuilder("select XXX,XXX,XXX");
StringBuilder sql = new StringBuilder("select ");
//通过 类加载器 读取文件
InputStream inputStream = AnnotationTest.class.getClassLoader().getResourceAsStream("bean.properties");
System.out.println(inputStream);//java.io.BufferedInputStream@7cca494b
//读取properties配置文件,获取到配置文件里指定的类名
Properties prop = new Properties();
prop.load(AnnotationTest.class.getClassLoader().getResourceAsStream("bean.properties"));
String className = (String) prop.get("className");
//使用反射通过类名加载类
Class<?> clz = Class.forName(className);
Table tableAnnotation = clz.getAnnotation(Table.class); //获取到类上的注解
String tableName = tableAnnotation != null ? tableAnnotation.value() : clz.getSimpleName().toLowerCase();
//使用反射获取类里的字段
Field[] fields = clz.getDeclaredFields();
for (int i = 0; i < fields.length; i++) {
//获取字段上的注解
Column columnAnnotation = fields[i].getAnnotation(Column.class);
//遍历字段,获取字段名,添加到SQL语句
String name = columnAnnotation != null ? columnAnnotation.value() : fields[i].getName();
sql.append(name);
if (i != fields.length - 1) {
sql.append(",");
}
}
sql.append(" from ").append(tableName);
System.out.println(sql);//select name,age,sex,city,score from t_student
}
}
@Table("t_student")
class Student {
private String name;
private int age;
@Column("sex")
private String gender;
private String city;
private String score;
}
@Retention(RetentionPolicy.RUNTIME)
@interface Table {
String value();
}
@Retention(RetentionPolicy.RUNTIME)
@interface Column {
String value();
}